Laravel is a free, open-source PHP web framework that has been under active development since 2011. It’s one of the most popular PHP frameworks, with a robust community of developers and a wide range of features. Laravel is designed to be fast and scalable, with built-in caching mechanisms that improve performance on larger applications. It’s also designed to be easy to use and includes a wide range of tools such as a command line interface (CLI) to quickly scaffold projects and features that make common web development tasks easier such as routing and authentication.
Application performance is especially critical for web applications, making Laravel a strong choice for web development. Users expect web applications to be responsive and fast, and slow page loads, errors, and other problems with your Laravel application can lead to lost customers and negatively impact your bottom line.
In this article, you’ll learn why it’s important for Laravel applications to be performant and how to monitor and improve the performance of your Laravel applications.
Why is Laravel performance optimization important?
The performance of your Laravel application is crucial for growing your user base, keeping your users happy, and growing your business. However, there are many factors that can negatively affect application performance, including poor code quality, inefficient server configurations, and insufficient resources. These issues can lead to slow loading times, errors, and crashes, so it’s important to monitor your application’s performance and make adjustments as needed.
In today's digital world, speed is everything. Users expect content to load quickly and smoothly, and they will quickly become frustrated if a site is slow or unreliable. This is especially true for e-commerce sites, where users need to be able to load pages quickly in order to browse and make purchases. If your application is slow to load, users will be likely to look for other applications that can better meet their needs. That’s why it’s especially important that you effectively use Laravel to build fast, responsive websites.
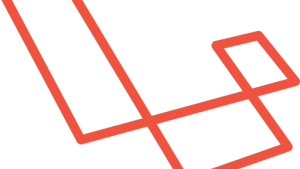
How to measure Laravel performance
Measuring the performance of a Laravel application involves a combination of strategies, among which utilizing monitoring tools stands out as a highly effective solution. Tools like New Relic, provide detailed insights into various aspects of the application, such as execution time, memory usage, database query efficiency, and response times. These tools enable developers to identify performance bottlenecks, inefficient queries, and code paths that need optimization. For instance, New Relic offers real-time monitoring and detailed analytics that help in understanding the application's behavior under different conditions. By implementing these tools, developers can gain a comprehensive overview of performance across all components of their Laravel application, ensuring that it operates efficiently and effectively.
So how can you optimize your Laravel performance? Let’s look at some best practices, ranging from basic improvements, such as updating your PHP version, to setting up tools to monitor the performance of both your Laravel application and your SQL database.
Update to the latest versions of PHP and Laravel
The first way to improve the performance of your Laravel application may seem a bit obvious—keep your PHP and Laravel versions up to date. While this can be more challenging with large legacy applications, using the latest versions gives you a number of benefits, such as new features, bug fixes, security patches, and optimizations that can improve the performance of your code.
- To check your current version of PHP: php -v
- To check your current version of Laravel, run the following command from the root directory of your project: php artisan --version.
Debug and optimize with Laravel Debugbar.
Laravel Debugbar is a cornerstone package for Laravel and provides detailed information about your application's execution time, memory usage, and database queries. Laravel Debugbar has a profiler enabled by default. This tool can identify which parts of your code are taking the longest to execute, helping you to focus your optimization efforts on those areas.
- Install the Debugbar with the command composer require barryvdh/laravel-debugbar --dev
- The Debugbar will be enabled when APP_DEBUG is true.
Configure Laravel’s built-in error handler
Laravel automatically provides error and exception handling when you bootstrap a new project. You can also customize error handling and the messages errors return, which can be especially useful for areas of your application that are mission critical or prone to issues. You should also ensure that you’ve configured log stacks on the backend to capture key events that lead up to the exception. This will help you reproduce the error and correlate events in monitoring tools such as New Relic.
Consider using Laravel Octane
Laravel Octane improves application performance by using powerful application servers. Octane boots your application just once and keeps it in memory, making it super fast. It also leverages a cache driver to improve read and write speeds.
Utilize route caching
Laravel loads all the routes defined in an application when it starts up. You should cache routes in a production application with the command php artisan route:cache. Without caching, Laravel needs to parse all of the routes in your application and this takes longer when your application has a lot of routes. With caching, Laravel simply needs to refer to the file where cached routes are stored.
Use database caching
You can cache popular database queries, reducing the load on your database, the server, and the client. Instead of hitting the database, Laravel can simply return the cached version of a query. This can provide a huge performance boost, especially for high-traffic sites and APIs. It can take some of the load off of the database itself, which can further improve performance.
Use Laravel queues
By offloading heavy tasks to a queue, you can free up resources on your web server and improve page load times. There are several ways to configure queues in Laravel, and the best approach will vary depending on your needs.
First, make sure to configure your queues for concurrency. This will allow multiple workers to process items from the queue simultaneously, which can greatly improve Laravel performance. Second, monitor your queues carefully. This will help you identify any bottlenecks or issues that may be affecting performance. Finally, remember to gracefully restart your queues during your deployment process using php artisan queue:restart. Queues are long-lived processes and they won't respond to changes in your application code without being restarted. To avoid having to restart manually, leverage a process manager like Supervisor to automatically restart queue workers.
Generate a class map with Composer
If you're building a Laravel project, one of the first things you'll do is use Composer to install dependencies. However, Composer can also be used to manage your codebase. By keeping your project's codebase in a separate directory from your dependencies, you can optimize Composer to improve performance. This is accomplished by generating a class map, which compiles your project's classes into a single file. With a class map, Composer doesn’t need to scan through multiple directories when loading classes, improving Laravel performance. In addition, class maps can help you better encapsulate your code by making it clear which files belong to your project and which files are dependencies. As a result, optimizing Composer can have a significant impact on the performance of your Laravel project.
Leverage dependency injection
One of the reasons for Laravel’s popularity is its use of dependency injection, which can improve performance by decoupling code modules and allowing them to be injected into different parts of the application as needed. This improves code reusability and testability and helps reduce the overall complexity of your codebase. In addition, dependency injection can also make it easier to manage dependencies between different libraries and frameworks.
Monitor and optimize database transactions
Review your Laravel application for any raw queries using DB::raw as raw queries can result in SQL injection vulnerabilities. While Laravel includes Eloquent ORM and Query Builder, which make it easier to write queries, you'll still need to watch for issues such as n+1 queries and lazy loading object relationships that aren't being used in certain routes. And when you need to optimize large queries for speed, consider using Query Builder instead of Eloquent as Query Builder is both faster and uses less memory.
New Relic includes quickstarts for MySQL, PostgreSQL, SQLite, and SQL Server, the four SQL databases that Laravel supports. These quickstarts provide key information, such as how many rows are being created, updated, and deleted per second.
Use rate limiting
Like all web applications, Laravel performance can be hindered by heavy traffic or malicious users. Rate limiting can be used to control the amount of traffic that your application receives, which can help improve Laravel performance and also protect your application from denial-of-service attacks. When configuring rate limiting in Laravel, you need to decide what kind of limit you want to impose, such as the total number of requests or the number of requests per IP address. You also need to decide how your application will handle requests exceeding the limit, such as returning an error or delaying responses. You can use a monitoring tool like New Relic to determine a rate limiting threshold for when your application performance starts to degrade.
Set up baseline performance monitoring and proactive alerting
Setting up alerts proactively empowers your engineering teams to catch issues before they become major incidents. With New Relic, you can instrument your Laravel application and set up monitoring in minutes. The Laravel quickstart helps you install the New Relic PHP agent and automatically get a prebuilt dashboard that provides an overview of key indicators such as transactions and errors, with information about CPU, memory, and disk space to provide further context. The quickstart also includes predefined alert conditions across application golden signals (including transaction duration, error rate, and throughput).
Benefits of Laravel performance optimization
Laravel applications are exceptional tools for your business. There are several benefits to keeping Laravel updated and optimized, a few of which are explained below.
- Improved user experience: Websites and applications will be able to handle more website traffic without lags, creating a better user experience for customers.
- Faster content delivery: Laravel clears caches and optimizes queries to speed up content delivery and reduce page load times.
- Increased business revenue: With a faster, smoother website or application, your target audience will be less inclined to leave your page. Optimizing your Laravel apps also saves money by boosting server efficiency without paying for upgrades.
- Reduced workload: An optimized Laravel app is easier to upkeep and preemptively addresses potential issues, reducing internal workload.
Using New Relic to improve Laravel performance
Laravel is a popular PHP framework that is known for its efficiency and flexibility. However, Laravel applications can sometimes experience performance issues—even when you are incorporating best practices and constantly improving your code. That’s where using an application performance monitoring (APM) tool like New Relic comes in.
An APM tool provides continuous real-time data and context about key performance metrics in your application such as HTTP request and response times, error rate, and throughput. In the case of New Relic, you can start monitoring your Laravel applications in minutes with Laravel instant observability. In addition to a prebuilt dashboard for key application metrics, you automatically get alerts for critical benchmarks in your application such as when the total error rate gets too high. With a combination of monitoring and alerts, you can quickly pinpoint and fix issues before they impact your customers. You can also use APM metrics to evaluate updates to your production code, allowing you to optimize your application’s performance further.
Next steps
- Sign up for New Relic free to get started.
- Monitor your Laravel applications in minutes with Laravel instant observability quickstart.
- Take a deeper dive into New Relic’s PHP documentation.
The views expressed on this blog are those of the author and do not necessarily reflect the views of New Relic. Any solutions offered by the author are environment-specific and not part of the commercial solutions or support offered by New Relic. Please join us exclusively at the Explorers Hub (discuss.newrelic.com) for questions and support related to this blog post. This blog may contain links to content on third-party sites. By providing such links, New Relic does not adopt, guarantee, approve or endorse the information, views or products available on such sites.