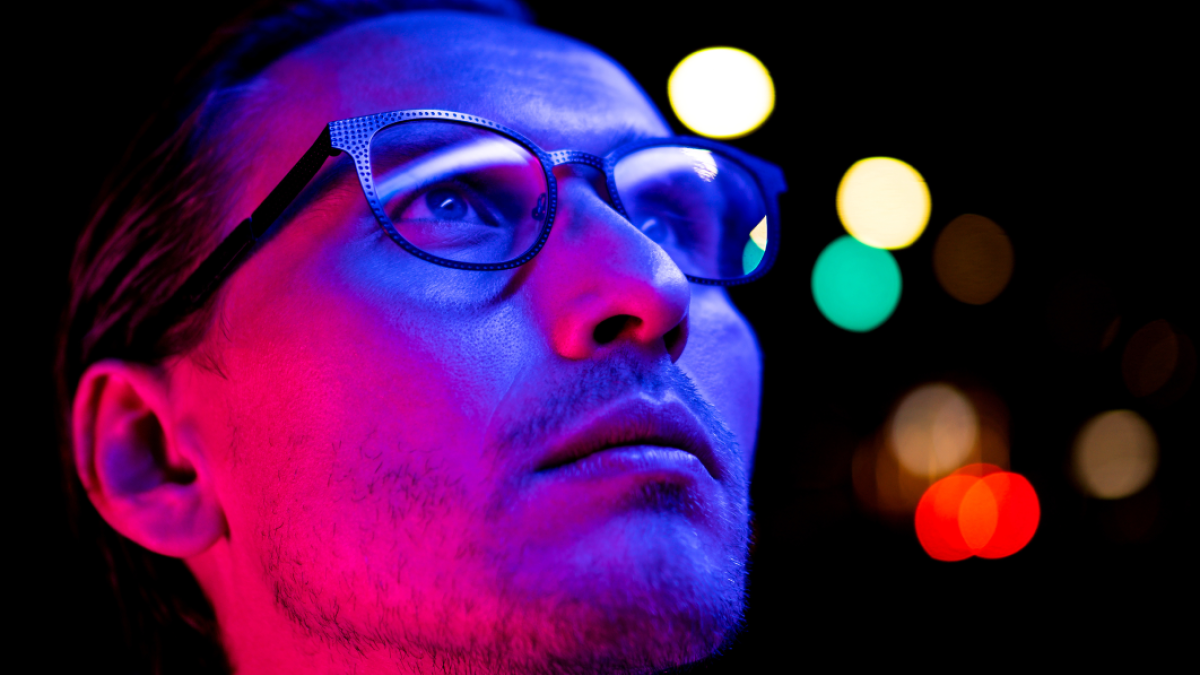
近年はセキュリティリスクの増大に伴い、パスワードだけではなく複数の要素で認証するWebサービスが増加しています。
Synthetic Monitoringは基本的な設定でも多岐にわたるチェックが可能ですが、全ての環境を網羅するにはより踏み込んだ活用が必要になります。
本記事ではセキュリティが強化されたサイトに対し、簡単なサンプルコードをもとに定期的に外形監視を実行する方法を解説します。
多要素認証とは?
まずは、多要素認証についての簡単に振り返ってみましょう。
wikipediaより:
多要素認証(たようそにんしょう、英語: Multi-Factor Authentication、英: MFA)は、アクセス権限を得るのに必要な本人確認のための複数の種類の要素(証拠)をユーザーに要求する認証方式である。
ID/パスワードだけではなく、Google AuthenticatorをはじめとするスマートフォンアプリやSMS、メールなどに対し発行されたトークンと合わせて認証する形がよく知られています。
本記事では、スマートフォンアプリなどで使用される複数桁の数字で構成されるトークンを利用した設定について解説します。
※本記事内に記載されているサンプルコードは運用の仕方によってはサービスに影響を及ぼす可能性があります。ご自身で提供されているサービスもしくは、監視・管理を委託されているなど管理する権限のあるサービスに限って実施してください。また、サービスの仕様によっては機械的なログインが繰り返し行われたり複数回ログインに失敗するとサービスから遮断されるといったことが起こり得ます。ご自身のアカウントがロックされたりしないよう、十分にご留意ください。
Syntheticsにおける多要素認証の扱い
Synthetic monitoringでは複数のタイプのmonitorを作成し、外型監視を行うことができます。そのなかでもScripted Browserではscriptを書くことでより環境に合った監視を柔軟に作成することができます。本記事ではScripted BrowseでOTPAuthパッケージを使い多要素認証環境の外型監視を実現しています。
設定例
多要素認証によるトークンの取得方法はGitHubにてサンプルが公開されています。
https://github.com/newrelic/quickstarts-synthetics-library/blob/main/library/2FAOrMFA/script.js
設定例では実際にサンプルコードを組み込み、ID/パスワード/多要素認証が設定されたページで認証に成功するまでのシナリオを解説します。
1. アプリケーション用の秘密鍵を取得する
OTPAuthは認証アプリの代替として動作しますが、複数桁のコードを生成させるためには秘密鍵が必要になります。 ここでは監視対象のサイトより秘密鍵を取得します。 ※セキュリティ上問題ないようであれば、検証のためご自身のデバイスを登録しておきましょう。
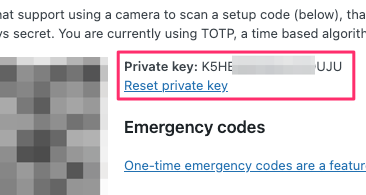
2. SyntheticsのSecure Credentialsに認証情報を保存する
Secure credentialより、画面右上のボタンより「Create secure credential」を選択してサイトログイン用のID/Passと手順1で取得した秘密鍵をそれぞれSecure Credentialsに登録します。
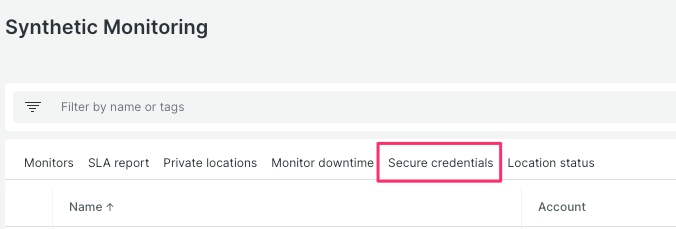
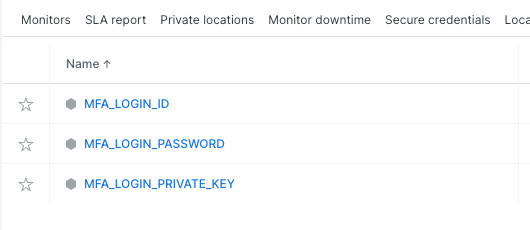
3. Scripted browser Monitorを作成する
User flow / functionalityを選択し、Scripted browserを作成します。
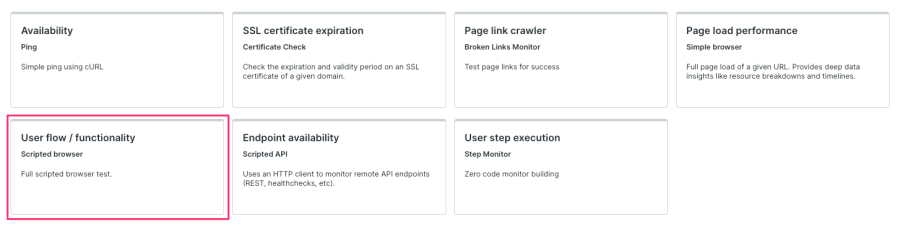
4. Monitorの設定を行う
OTPAuthパッケージを利用する要件として、Chrome 100以上、Node 16.10.0以上が要求されます。 Runtimeは忘れずに Chrome 100+ を選択しましょう。 Locationはご利用される方の環境に合わせてご選択ください。
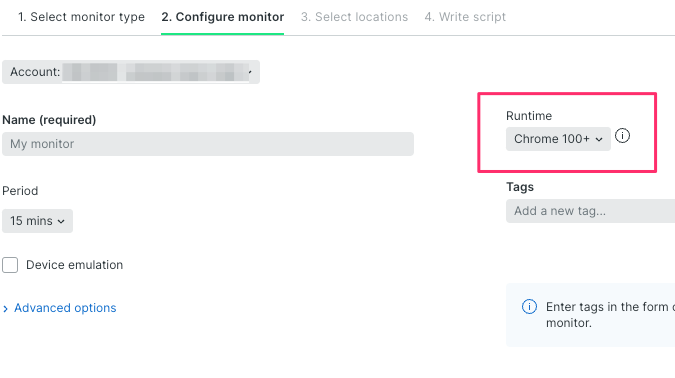
5. Scriptを書く
それでは実際にscriptを書いてみましょう。 scriptの構成としては下記の流れとなります。
- 対象サイトを開く
- ログインIDを入力
- パスワードを入力
- 決定ボタンを押しログイン
- 多要素認証トークン要求画面への遷移を待つ
- 多要素認証トークン要求画面でトークンを入力
- 決定ボタンを押してログイン
- マイページへの遷移を待つ
手順5では実際に秘密鍵をもとにTOTP (Time-based, One-Time Password) を利用したトークンを生成、入力ボックスにトークンを入力しています。
※Time-basedとありますように、scriptの先頭部分でトークンを取得すると実行時間が長引いた際にトークンが失効する場合があります。
できる限り、トークンを要求される画面に近い場面でトークンを生成しましょう。
まとめ
Scripted browserではパッケージを利用た認証方法の追加をはじめとして、さまざまなモニタリングを構成できます。
また、今回ご紹介させていただきました多要素認証対応の他にも様々なサンプルが公開されています。
Simple browserなどSyntheticの他の機能では実現が難しい環境などでご利用にお悩みでしたら、より柔軟に設定可能なScripted Browser利用の足がかりになりますと幸いです。
本ブログに掲載されている見解は著者に所属するものであり、必ずしも New Relic 株式会社の公式見解であるわけではありません。また、本ブログには、外部サイトにアクセスするリンクが含まれる場合があります。それらリンク先の内容について、New Relic がいかなる保証も提供することはありません。